How to deploy a SvelteKit app to Heroku
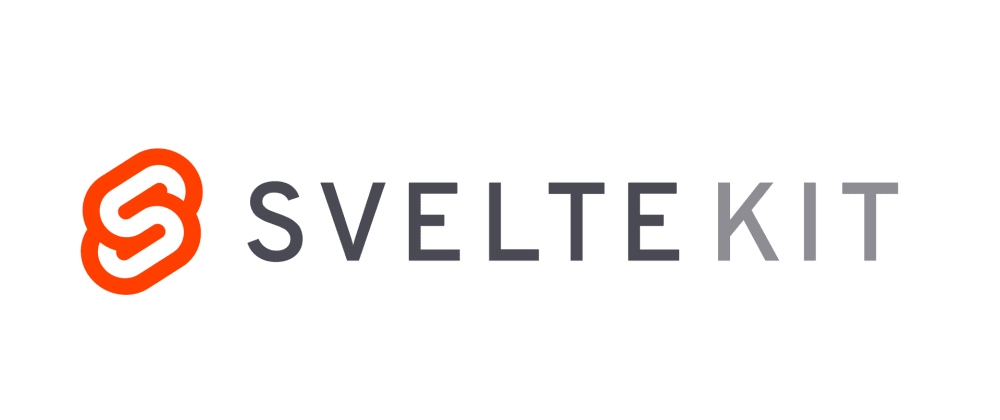
Another day, another interesting little bit of knowledge. This time, it’s how to deploy a SvelteKit app to Heroku.
SSR Vs Static
SvelteKit is, by default, a server-side rendered framework. This means that when you request a URL, the server will render a page and send it back to the user as HTML. If you’re used to Django, Flask, Rails or any other MVC style framework this will make total sense to you. However, most React / Vue apps are totally client side rendered. This means that the client (i.e. the browser) will request a page and the server will send back a bunch of JavaScript. This JS is then used to render the page.
Advantages of SSR:
- Better SEO
- Faster initial load times
- Better accessibility
- You can have actual links to pages
However, this means you have to have an actual server running somewhere to render the pages. For my set-up i’ve got a Go backend running on Heroku and I want to deploy my SvelteKit app to Heroku too. Here’s how you get a SvelteKit app deployed to Heroku.
Note: This tutorial assumes you’ve already got a SvelteKit app and a Heroku account.
Step 1: Configure your build adaptor.
In your SvelteKit app, you’ll need to configure your build adaptor. This is what tells SvelteKit how to build your app. Firstly, install the node adatpor:
1
npm install --save-dev @sveltejs/adapter-node
Then, in your svelte.config.js
file, add the following:
1
2
3
4
5
6
7
8
9
10
11
12
13
import adapter from '@sveltejs/adapter-node';
import { vitePreprocess } from '@sveltejs/vite-plugin-svelte';
/** @type {import('@sveltejs/kit').Config} */
const config = {
preprocess: vitePreprocess(),
kit: {
adapter: adapter()
}
};
export default config;
Step 2: Edit Your package.json
You’ll need to add a start script to your package.json file. This is what Heroku will use to start your app.
1
2
3
4
5
6
{
"scripts": {
"build": "vite build",
"start": "node build"
}
}
Step 3: Deploy to Heroku
Now you’ve got your app configured, you can deploy it to Heroku. Firstly, you’ll need to create a new Heroku app. You can do this from the Heroku dashboard or from the command line. Honestly, I’d recommend the UI.
- https://dashboard.heroku.com/apps - Click ‘New’ and then ‘Create new app’
- Then go to the ‘Deploy’ tab and connect your GitHub account.
- Search for your repo and click ‘Connect’
- Click ‘Enable Automatic Deploys’
- Click ‘Deploy Branch’ and wait for the build to finish.
- Click ‘View’ to see your app.
That’s it, job done. You’ve now got a SvelteKit app deployed to Heroku.